This tutorial describes the overall software architecture of ResearchPackage and its API, and how to get started.
Architecture and API
API Naming
We are following the API naming pattern from Apple’s Research Kit. ResearchKit uses the prefix ORK
(Open Research Kit) before the names of classes, types etc. Following this naming model, the class names in ResearchPackage starts a RP
prefix. UI widgets uses the RPUI
(Research Package UI) prefix.
> Note that objects prefixed with 'RP' are part of the Model (e.g.RPConsentDocument
) while those prefixed with 'RPUI' are part of the UI library (e.g.RPUIVisualConsentStep
).
Domain Model
At the top, ResearchPackage uses a Task
to hold the individual steps the user must go through, fx. a series of questions or an informed consent with a few sections of information to read about. The task models available are RPOrderedTask
and RPNavigableOrderedTask
. Both are ordered but the latter can navigate inside the questions if needed.
A step can be either RPQuestionStep
, RPInstructionStep
, RPFormStep
, RPCompletionStep
, RPVisualConsentStep
or RPConsentReviewStep
, depending on what is needed. The latter two are used for consent only, but it is also possible to mix them with the other steps.
For the RPNavigableOrderedTask
, ResearchPackage uses RPStepNavigationRule
types to specify any behavior where the task should not simply go to the next step every time. The different types of navigation rules are: RPStepJumpRule
, RPStepReorganizerRule
and RPDirectStepNavigationRule
. An example of a navigation survey could be the situation below:
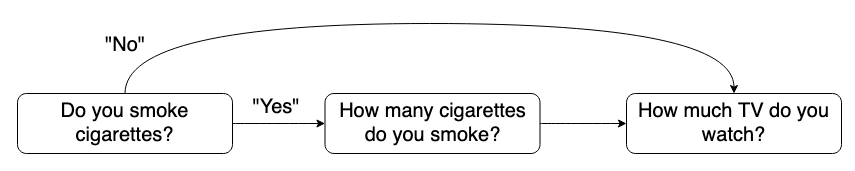
In the example below, a survey is modelled as a task with 5 steps. The task is an RPOrderedTask
object and the steps are RPQuestionStep
with and RPInstructionStep
after the first question. Each RPQuestionStep
is created with an RPAnswerFormat
which decides how the the question can be answered.
Once the task is “done”, the answers (results) are saved as a RPTaskResult
object.
BLoC Architecture
ResearchPackage follows the BLoC architecture, which is recommended by the Flutter Team. In ResearchPackage, the BlocTask
is holding the StreamControllers
needed for the communication. Following the BLoC architecture, ResearchPackage uses streams and callbacks for internal communication (e.g. between Task and Steps). There is a BLoC class responsible for the communication between the task and the steps it’s containing (BlocTask
). Communication between the question container and their question body is made possible via callback functions.
Style and UI Theme
Research Package is accessing the parent application’s style through Flutter’s context
feature. This means that the given Theme
of the application is shared also with Research Package and appears in its styling (colors, font styles…). As an example, the example application shows how the theme colors of the parent application are modified, and this is reflected in the ResearchPackage screens. See this article by the Flutter team on how to Theme your application.
ResearchPackage uses the values of specific Theme
-items such as the Theme.of(context).textTheme.headline6
for styling, an as such it is possible to parse a TextStyle
to the theme and with the ability for complete customization. Some other used theme-values are sliderTheme.activeColor
, sliderTheme.inactiveColor
, textTheme.subtitle1
and colorScheme.onPrimary
. Many more are used and you may consult the source code for specific elements.
Here is an example of how to change the these default values:
Widget build(BuildContext context) { return Theme( data: ThemeData.light().copyWith( sliderTheme: SliderThemeData( activeTrackColor: Colors.green, inactiveTrackColor: Colors.red, )), child: RPUITask( ... ), ); }
How to set up a ResearchPackage project
Research Package is published to `pub.dev` and can be add as a dependency in the pubspec.yaml
file.
dependencies: research_package: ^{latest_version}
After you run the flutter packages get
command, you are able to import and start using ResearchPackage. You can import the whole package including the Model and UI libraries:
import 'package:research_package/research_package.dart';
Or you can decide which library of the package you want to use:
import 'package:research_package/model.dart'; import 'package:research_package/ui.dart';
Answer Formats
Answer formats are extension on the RPAnswerFormat
class. They all change how the RPQuestionStep
can be answered depending on which answer format is chosen. The answer formats currently available are:
- Singe Choice
- Multiple Choice
- Integer
- Date and Time (Date)
- Date and Time (Time of Day)
- Date and Time (Date and Time)
- Image Choice
- Slider
- Free Text
- Form Step (This answer format is not used, but will be automatically applied if using a
RPFormStep
)
Below is a UI representation of the currently available answer formats of Research Package at the moment.
Single Choice | Multiple Choice | Integer
Date And Time (3 answer styles)
Date Answer Type | Date and Time Answer Type | Time of Day Answer Type
Image Choice | Slider | Free Text
Form Step
It is possible to put multiple questions on the same page by using a form step – RPFormStep
. Each question step have a separate card, which is shown below.
Active Tasks
Research Package has a sister package Cognition Package that builds upon the active tasks of Research Package.
Limitations and Contributing
ResearchPackage currently supports the answer formats listed above, which can be put on separate pages or combined in one page (using a FormStep
). Nonetheless, we are constantly working on the package so these features, as well as other types of questions will be implemented in the future.
ResearchPackage is available as open source under an MIT license, so if you’re interested in helping to maintain and expand it, please reach out and make pull requests. Also, please report any issues you may find.